So let’s talk CSS selectors – these little guys are the unsung heroes of web design trust me.


I’ve seen enough websites built with and without them to know the difference my friend.
Think of them as the secret weapon for tweaking and styling your web pages.
Without them you’re basically wrestling a greased pig in the dark.

The Universal Selector: The Big Kahuna
This bad boy represented by an asterisk (*) selects EVERYTHING on the page. Yep every single element. It’s like wielding a nuclear option – powerful but use it sparingly. Imagine trying to style every single element individually; you’d be pulling your hair out faster than a cat in a yarn factory! The universal selector gives you the power to do things like set a default font or apply a base style to your entire site. It’s efficiency on steroids. However be warned: overuse can lead to a cascade of unintended consequences making your CSS a total nightmare. I’ve learned this the hard way; there are many times I regret using this selector and there are better ways to accomplish many of the tasks it is useful for. Remember with great power comes great responsibility (and maybe some serious headaches).
Universal Selector Gotchas and Best Practices
Using the universal selector incorrectly can lead to performance issues especially on large websites with many elements therefore you should only use it when necessary.
It can make your code bloated and slow down loading times a cardinal sin for any website.
Check our top articles on CSS Selectors: Diese Selektoren sollten Sie unbedingt kennen
Furthermore it can be difficult to track down unforeseen style conflicts down the road.
Your future self will thank you for a well-organized and selective application.
My advice: only use it if you have a very good reason which usually involves applying styles to absolutely every element.
For instance you might use it to reset default browser styles but even then a more targeted approach might be preferable.
There are plenty of times I’ve wished I could go back and refactor my code to avoid the universal selector.

I’ve seen it happen time and time again; junior developers eager to show off their skills unleash the universal selector with reckless abandon.
Suddenly the entire website is awash in unintended styles.
Debugging this kind of thing is like searching for a needle in a haystack – you’re gonna need a lot of patience coffee and maybe a little bit of luck.
Trust me I’ve been there.
My suggestion is to approach it carefully.
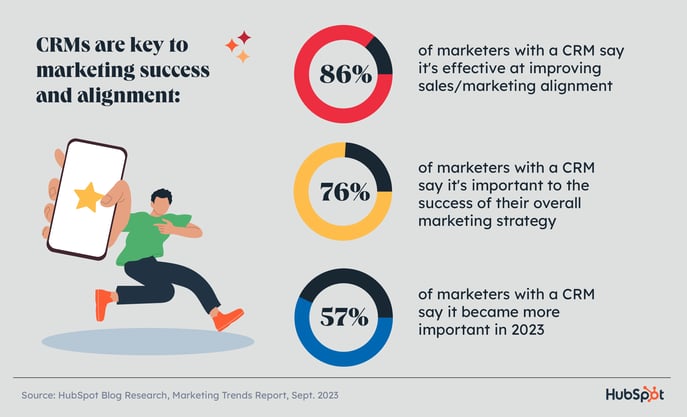
It’s a powerful tool but it’s best used as a last resort not a first choice.
Always explore more targeted selectors first – you’ll thank me for it.
Type Selectors: Targeting by Element Name
These are your everyday workhorses.
They select elements based on their HTML tag name like <p>
for paragraphs or <h1>
for headings.
For example p { color: blue; }
turns all paragraphs blue.
Simple effective and your best friend for basic styling.
However if you find yourself repeatedly styling the same type of element in different ways it might be time to consider CSS classes or IDs for more precise control.
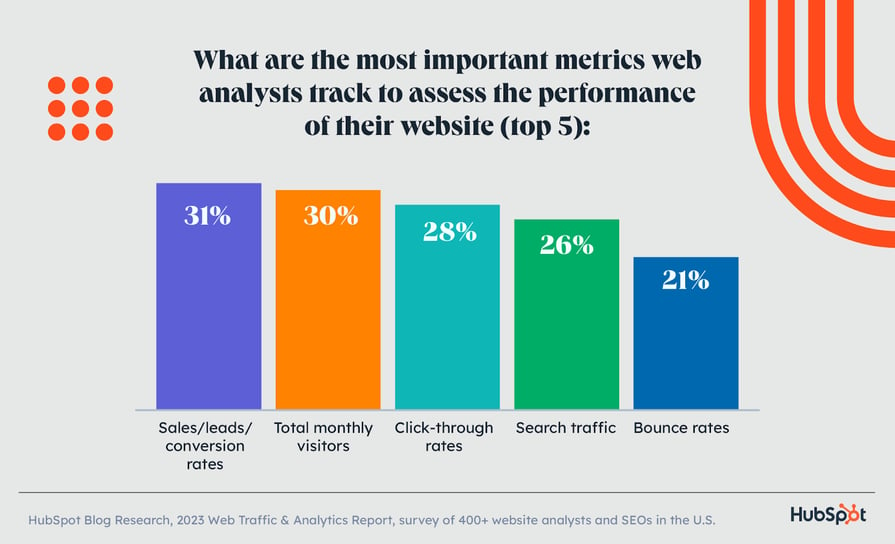

Beyond the Basics: Nesting and Specificity
What happens when you have nested elements? You can absolutely target the nested elements.
For instance div p { font-size: 14px; }
will only affect <p>
elements that are inside <div>
elements.
This is where things get interesting – and sometimes a little tricky.
CSS uses a system of specificity to determine which style rules take precedence when they conflict.
In general rules with more specific selectors override more general ones.
You can have a lot of fun playing with this but it also comes with the risk of creating a frustrating conflict – one that makes debugging seem like an impossible mission.
Specificity is an important concept to master.
It can feel like a tangled web especially when dealing with nested elements and multiple selectors.
Think of it as a hierarchy of style rules – the more specific a selector is the more power it holds.
A rule targeting a specific element with a specific class will override a more general rule that targets the element type only.
It’s like a game of rock paper scissors but with style sheets! Learn it well because a poor understanding of specificity will lead to all sorts of unpredictable behavior.
Class Selectors: Adding Granularity
Class selectors let you add classes to your HTML elements and then style those specific classes.
For instance <p class="intro">
allows you to apply unique styles just to that paragraph.
That’s where the .intro { font-style: italic; }
magic happens.
This adds a layer of control not possible with type selectors alone.
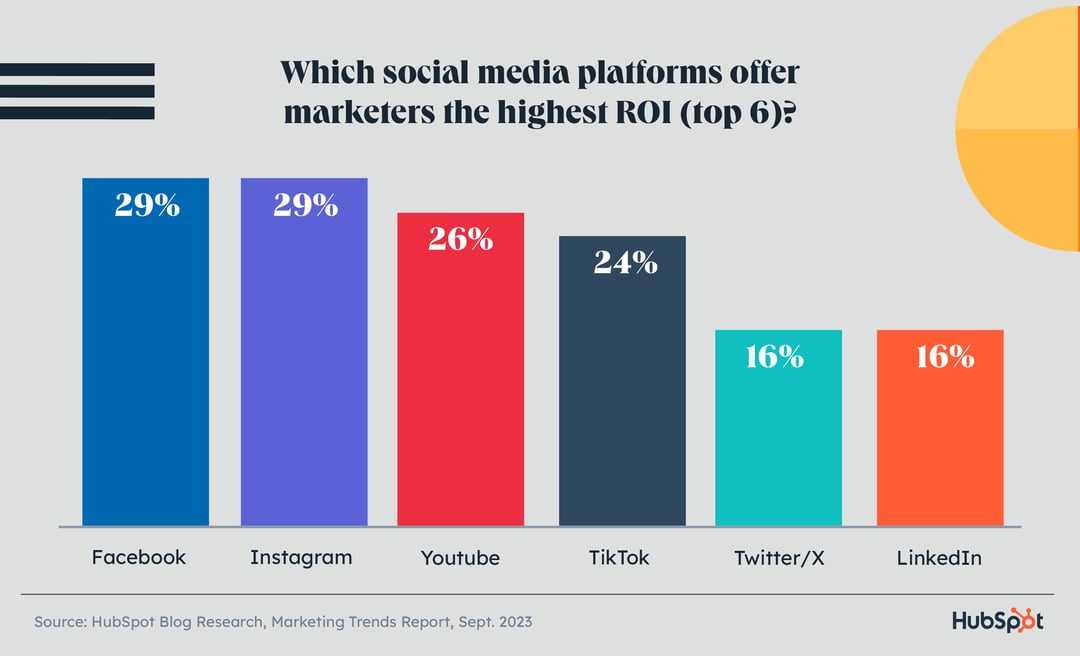

I’ve always found this to be a fundamental part of good CSS structure; they give you such a clean and well-structured workflow.
Class Selectors and Reusability
The beauty of class selectors lies in their reusability.
Once you’ve defined a class you can apply it to multiple elements scattered throughout your HTML.
This leads to DRY (Don’t Repeat Yourself) code saving you time and effort.
Imagine needing to change the styling of a specific element type across multiple pages you wouldn’t need to edit them individually.
Instead you make the change in one place – the class selector and it cascades to all the instances of that class.
It’s a huge time saver.
There’s nothing worse than having to update the same style in numerous places.
I’ve seen plenty of people who initially resist class selectors.
They prefer to use inline styles or overly specific selectors making their CSS convoluted and unwieldy.
But once you get used to class selectors you’ll find that they’re an indispensable part of your CSS toolkit.
They simplify your code and make it maintainable especially as your project scales.
My advice – embrace this powerful tool and be amazed at the structure it brings to your CSS.
ID Selectors: Unique Identifiers
ID selectors like class selectors target specific elements. The key difference is IDs must be unique on the page. Each element should only have one ID. This is crucial for accessibility and performance. They start with a #
like #main-content { width: 80%; }
. I tend to use these sparingly mostly for things that need to be uniquely identified like a main content area or a modal dialog.
IDs vs. Classes: When to Use Which
The choice between ID and class selectors often depends on the intended use.
IDs should only be used when an element needs a unique identifier either for JavaScript interactions or styling elements that absolutely must be unique.
Level up your web dev game! 🚀 Want to wield CSS selectors like a pro? This guide’s got you covered. Check it out, you won’t regret it 😎
Classes on the other hand are perfectly fine to reuse across different elements making them better suited for general styling.
Mixing them up can lead to unpredictable styling results and is generally bad practice.
I’ve had many opportunities to learn this the hard way.
For instance I once used an ID where a class would have been more appropriate.
The result? A complete nightmare to debug.

The CSS conflicted with itself leading to some elements styled unexpectedly.
Remember ID selectors are for unique identifiers only.
Classes are much more versatile.

There is no need to overuse IDs; keep it simple and you’ll be well on your way.
Attribute Selectors: Getting Specific
Attribute selectors allow you to target elements based on their attributes. For example { ... }
styles all checkbox inputs. You can get even fancier with operators like ~=
(contains) |=
(begins with) and *=
(contains). They provide a finely-grained approach for selecting specific elements especially when dealing with dynamic content.
The Power of Attribute Selectors
These can be really handy when you need to style elements based on their attributes.
You might use them to style links based on their target attribute or to style elements based on custom data attributes.
This is especially powerful with JavaScript allowing you to dynamically add and remove styles based on conditions.
It’s like the advanced option of selecting elements; a more robust approach compared to the simpler type selectors.
I’ve used these extensively when working with complex forms.
I use them to apply different styles to input fields based on their type data attributes or even the values they are assigned.
In that case it became a lifesaver in ensuring that the input fields are correctly styled and that the user interface is consistent.
Attribute selectors give you flexibility and precision but remember to use them judiciously to avoid overly complex CSS.
Don’t let them become too abstract.
Pseudo-classes and Pseudo-elements: Beyond the Basics
Pseudo-classes and pseudo-elements give you access to states and parts of elements that aren’t directly represented in the HTML.
Pseudo-classes like :hover
:focus
and :active
style elements based on user interaction.
Pseudo-elements like ::before
and ::after
allow you to insert content before or after an element.
They are truly remarkable extensions to the core selector functionality.
They enable some seriously creative stuff but you need a bit of finesse.
Mastering Pseudo-Classes and Elements
I must admit that I initially found these confusing but once you get a hold of it you’ll be able to generate some seriously cool effects.
You can create interactive effects highlight elements based on states and add decorative elements without needing to alter the underlying HTML.
For instance you can use :hover
to change the color of a link when the user’s mouse hovers over it.
This kind of enhancement improves user experience.
However it takes practice.
I’ve seen many people struggle with pseudo-classes and elements initially.
They can be a bit abstract and it’s easy to make mistakes but keep practicing and you’ll be building complex and dynamic styles in no time.
There are countless resources available online.

Don’t be afraid to experiment; that’s the best way to learn!
Combinators: Connecting the Dots
Combinators let you combine selectors to target elements based on their relationship to each other.
The descendant combinator (` `) selects all descendants of a specific element.
The child combinator (>
) only selects direct children.
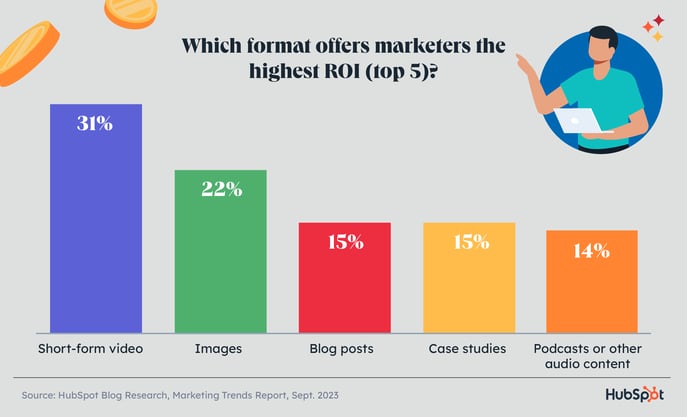
The adjacent sibling combinator (+
) selects the immediately following sibling.
And the general sibling combinator (~
) selects all following siblings.

They provide a powerful way to target very specific sets of elements.
The Art of Combining Selectors
This is where the power of CSS really shines.

It allows you to build complex selectors that only select specific elements based on their relationship to each other and their attributes and classes.
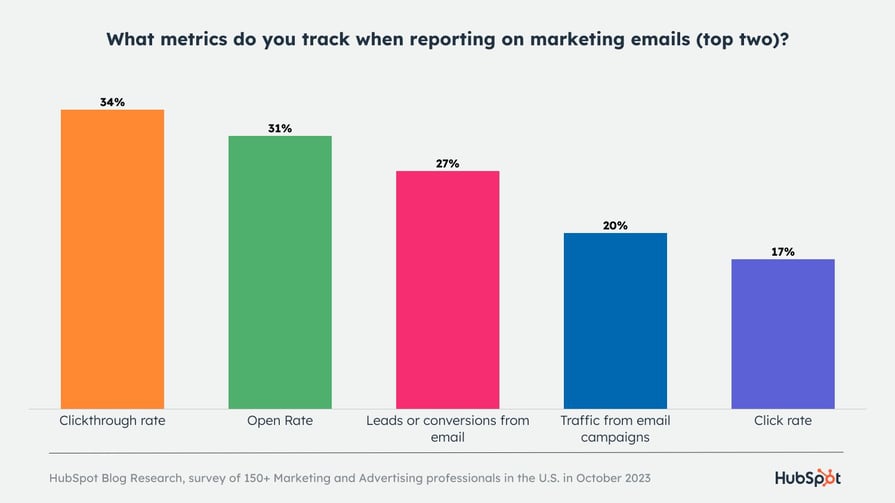
It takes practice but it’s well worth the effort.
With combinators you can create super-targeted styles that keep your CSS incredibly clean and well-organized.
I’ve used combinators extensively in my work.
I’ve seen others struggle with these ending up with overly complex and messy CSS.

But with practice you’ll become more adept at choosing the correct combinator for the situation.
This results in far more efficient and maintainable code.
In closing mastering CSS selectors is like gaining superpowers in web design.

It’s a journey not a destination.
It takes time practice and a willingness to experiment.
Level up your web dev game! 🚀 Want to wield CSS selectors like a pro? This guide’s got you covered. Check it out, you won’t regret it 😎
But the rewards are worth it.
So buckle up and let’s dive into the exciting world of CSS selectors! Remember don’t be afraid to make mistakes – we all do.
The important thing is to learn from them and keep building.
Level up your web dev game! 🚀 Want to wield CSS selectors like a pro? This guide’s got you covered. Check it out, you won’t regret it 😎
Now go forth and style!
